Automatically Publish Cryptocurrency Prices to Social Media Networks
Ayrshare makes it very easy to start publishing to your social media networks. One example is automatically publishing Cryptocurrency prices and changes, such as Bitcoin, Litecoin, or Ethereum. The code is available at GitHub.
There are three key parts: gather the prices, format the data, and publish to your social media networks.
Part 1: Gather Cryptocurrency Prices
There are numerous freely available APIs to get crypto prices. We choose Coin Gecko’s API since it didn’t require an API key and has an easy to use Node NPM package (also available in other languages, such as Python).
const CoinGecko = require("coingecko-api");
const CoinGeckoClient = new CoinGecko();
const crypto = await CoinGeckoClient.simple
.price({
ids: Array.from(["bitcoin", "litecoin", "ethereum"]),
vs_currencies: ["usd"],
})
.catch(console.error);
In the returned crypto object there is a data object of all the current prices of the coins we requested.
Part 2: Format the Data
Format the data so it presents nicely on your networks.
/** Publish the Crypto prices */
const coinMapping = new Map([
["bitcoin", "BTC"],
["ethereum", "ETH"],
["litecoin", "LTC"],
]);
const publishPrices = (data) => {
const keys = Object.keys(data);
const formatNumber = (num) =>
parseFloat(num)
.toFixed(2)
.replace(/(\d)(?=(\d{3})+(?!\d))/g, "$1,");
const json = {
post: `Hourly crypto prices:\n\n${keys
.map(
(coin) =>
`${coinMapping.get(coin)}: $${formatNumber(
data[coin].usd
)} (${coin}) ${data[coin].diff}`
)
.join("\n")}\n\n${keys.map((coin) => `#${coin}`).join(" ")}`,
platforms: PLATFORMS,
};
return publish(json);
};
Additionally, you can build special posts/alerts when there are significant price movement.
/** Publish if large price movement */
const coinMapping = new Map([
["bitcoin", "BTC"],
["ethereum", "ETH"],
["litecoin", "LTC"],
]);
const PLATFORMS = ["twitter", "facebook", "linkedin", "telegram"];
const publishMovement = (coin, percent, diff) => {
const json = {
post: `${coinMapping.get(coin)} (${coin}) is moving. ${
diff > 0 ? "Up" : "Down"
} ${percent}% in the past hour.`,
platforms: PLATFORMS,
};
return publish(json);
};
Part 3: Publish to Social Media Networks
The final step is publishing your posts to your networks. These posts can be real-time or scheduled, but for our use case we will do immediate publishing.
Ayrshare’s API facilitates connecting to all your social media networks and publishing via an API from your back-end/cloud functions. Go to Ayrshare, signup, and get your API key.
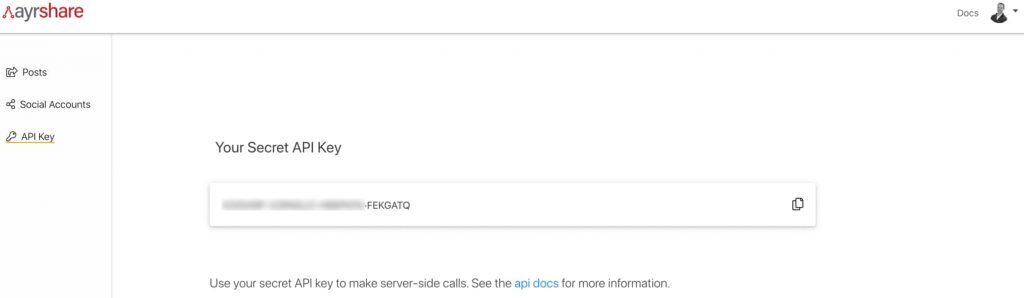
Then write your API call using your preferred HTTP request package (got, request, node-fetch) with your API key.
/** Publish to Ayrshare */
const publish = (json) => {
return got
.post("https://app.ayrshare.com/api/post", {
headers: {
"Content-Type": "application/json",
Authorization: `Bearer ${AYRSHARE_API_KEY}`,
},
json,
responseType: "json",
})
.catch(console.error);
};
All Done
That is it. You’ve published Crypto prices to your networks via an API. Now to run on a regular cadence, every hour, you need to setup a CRON job. We used Firebase as a platform and their scheduled cloud functions, but you can use the infrastructure of your choice.
exports.cryptoHourly = functions.pubsub
.schedule(CRONTAB_HOURLY)
.timeZone(TIME_ZONE)
.onRun((context) => {
return runHourly();
});
If you have any questions, drop us a line. Also, check out the code on GitHub for more details, code, and instructions.