Creating Social Media Posts with ChatGPT API
If you’re not living under a metaphorical technology rock then you’ve heard or tried ChatGPT. You know, the OpenAI freaky good chatbot. Since it was launched in November 2022, ChatGPT has been only available via the GUI interface – and when we say “available” we mean the OpenAPI advanced language model “gpt-3.5-turbo” that powers ChatGPT. Other, not as awesome, language models exist. However, recently OpenAI released their promised ChatGPT API that allows you to call the “gpt-3.5-turbo” via an API. This, dear friends, opens a world of new applications including ChatGPT created social media posts.
Why Use the ChatGPT API for Social?
There are a couple of reasons why you might want to integrate ChatGPT API into your platform or app:
User Create Content on Your Platform
Your users create content, such as images or videos, in your platform and share to the social networks, but a friction point is writing the social post content. For example, your creator marketplace produces an amazing image of a cat sitting in a shoe – you’re promoting the shoes, not the cat – and you have a button to share to the shoe-cat picture to Instagram.
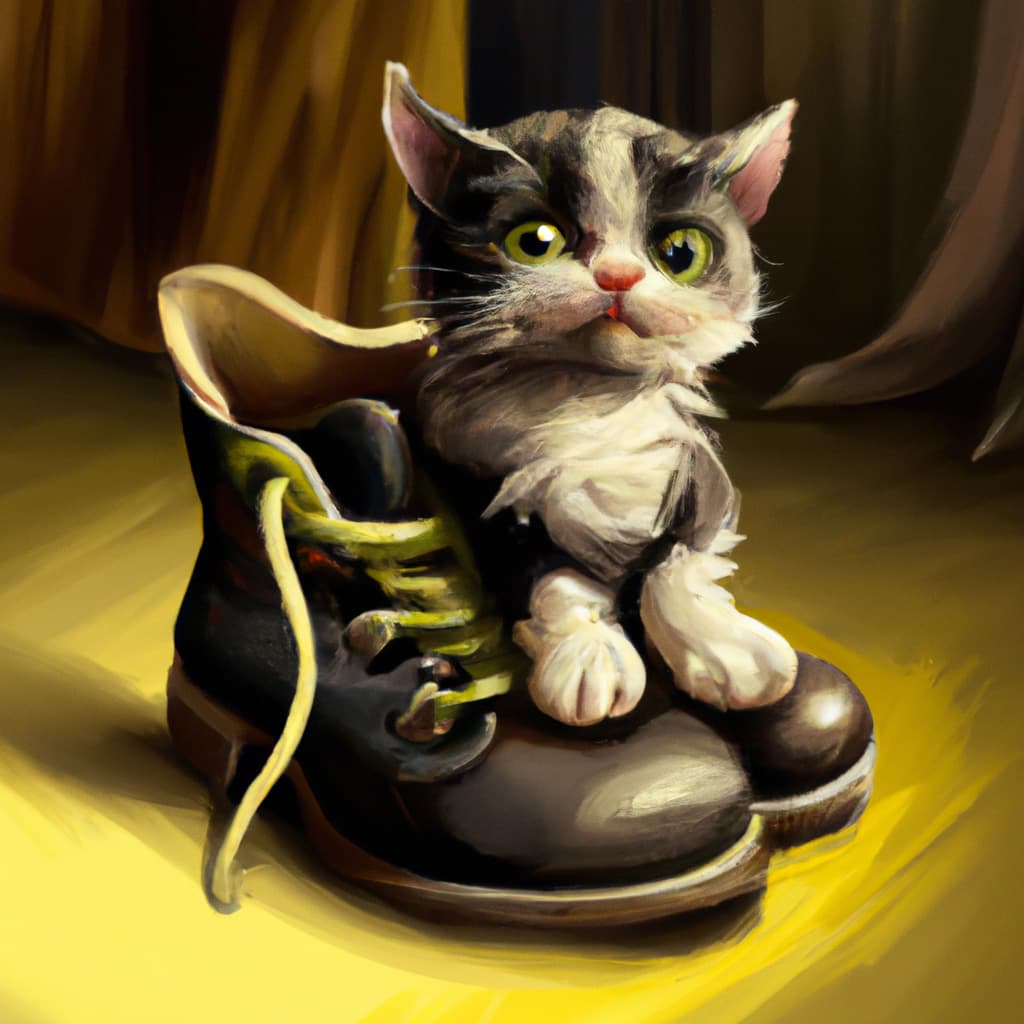
But what text do you want to add for that post, and what hashtags? Do you make the user write it or do you use templated text. Neither are good solutions.
ChatGPT is Hot Right Now
Everyone is talking about ChatGPT and integrating AI into your platform is the thing to do, and even gives some public companies a stock boost when the announce AI integrations. It might feel like following the crowd, but it truly is useful for your user and clients. If you can remove friction, increase client satisfaction, and possibly increase revenue, go for it.
Generating the Social Media Post Text using ChatGPT API
Let’s get into the fun stuff and generate a social media post with text from the ChatGPT API and post it to Instagram an Facebook using Ayrshare’s social media APIs. Note we will be using an an AI generated image using DALL·E.
Get a Chat API Key
The first step is to sign up for an OpenAI account and generate an API Key. Just click your profile icon in the upper right corner and select “View API Keys”.
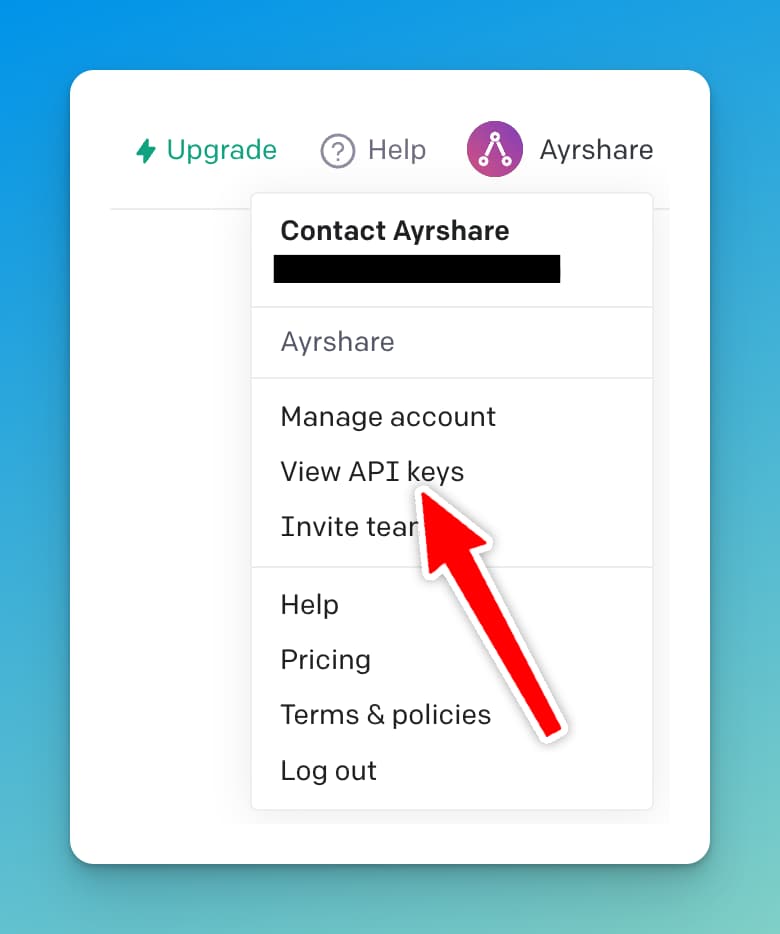
Signing up for Open AI is free and you get a generous amount of credits to try things out.
Call the ChatGPT API Endpoint
With our API Key in hand, let’s make our first API endpoint call. Every request to ChatGPT must have a request, such as “write me a blog post on the what the TV show Yellowstone is popular”. In our example, we’ll use the text “Write a social media post on a cat sitting in a shoe”. We’ll also use GPT-3 language model. However, we recommend using the latest language model, GPT-4, since it provides better results.
Here is a cURL example:
curl https://api.openai.com/v1/chat/completions
-H "Authorization: Bearer $OPENAI_API_KEY"
-H "Content-Type: application/json"
-d '{
"model": "gpt-3.5-turbo",
"messages": [{"role": "user", "content": "What is the OpenAI mission?"}]
}'
We’ll use the JavaScript examples going forward, but since these are REST calls you can write in the programming language of your choice, such as Python or PHP.
const OPENAI_API_KEY = "Your API OpenAI Key";
const run = async () => {
const options = {
method: "POST",
headers: {
Authorization: `Bearer ${OPENAI_API_KEY}`,
"Content-Type": "application/json",
},
body: JSON.stringify({
model: "gpt-3.5-turbo",
messages: [
{
role: "user",
content: "Write a social media post on a cat sitting in a shoe",
},
],
}),
};
const chatGPTResults = await fetch(
"https://api.openai.com/v1/chat/completions",
options
).then((res) => res.json());
console.log("ChatGPT says:", JSON.stringify(chatGPTResults, null, 2));
};
run();
What does this code do? Details guides can be found in the OpenAI docs, but here is the quick summery.
- POST calls to the URL
https://api.openai.com/v1/chat/completions
for ChatGPT API requests. Authorization
: Include the OpenAI API Key in the header.model
: Include in the body of the post the model name, which for ChatGPT isgpt-3.5-turbo
.role
: Eithersystem
,user
, orassistant
. We want to useuser
since we are asking for something.content
: The question or request we make to ChatGPT.
The result of the call:
{
"id": "chatcmpl-6ruxjLkNNtIKeZ0dPdCGG2ijXIYHd",
"object": "chat.completion",
"created": 1678308367,
"model": "gpt-3.5-turbo-0301",
"usage": {
"prompt_tokens": 19,
"completion_tokens": 56,
"total_tokens": 75
},
"choices": [
{
"message": {
"role": "assistant",
"content": "nnThis adorable little kitten has found a new favorite spot to lounge around in! Can you guess what it is? Yep, you got it- a shoe! How cute is this? 😍😻 #catinshoe #kittenlove #cutenessoverload"
},
"finish_reason": null,
"index": 0
}
]
}
The ChatGPT assistant answers in the content
field:
nnThis adorable little kitten has found a new favorite spot to lounge around in! Can you guess what it is? Yep, you got it- a shoe! How cute is this? 😍😻 #catinshoe #kittenlove #cutenessoverload
Not only is this a great summary, but we have emojis and even hashtags. If you call the endpoint again, you’ll get a different result.
Posting to the Social Networks
Now that we have our text for the post, let’s publish the post. We’ll be using Ayrshare’s social API and you can sign up for a free account to test. After signing up, get your Ayrshare API Key, last key you need to get, we promise, can connect some social accounts in the Social Accounts tab, such as Facebook, Instagram, and Twitter.
We’ll update our code to now take the generated text and post it to the social networks using the cat in a shoe image.
const OPENAI_API_KEY = "Your API OpenAI Key";
const AYRSHARE_API_KEY = "Your API Ayrshare Key";
const run = async () => {
const options = {
method: "POST",
headers: {
Authorization: `Bearer ${OPENAI_API_KEY}`,
"Content-Type": "application/json",
},
body: JSON.stringify({
model: "gpt-3.5-turbo",
messages: [
{
role: "user",
content: "Write a social media post on a cat sitting in a shoe",
},
],
}),
};
const chatGPTResults = await fetch(
"https://api.openai.com/v1/chat/completions",
options
).then((res) => res.json());
console.log("ChatGPT says:", JSON.stringify(chatGPTResults, null, 2));
const optionsPost = {
method: "POST",
headers: {
Authorization: `Bearer ${AYRSHARE_API_KEY}`,
"Content-Type": "application/json",
},
body: JSON.stringify({
post: chatGPTResults.choices[0].message.content,
platforms: ["facebook", "instagram"],
mediaUrls: ["https://img.ayrshare.com/012/cat-in-shoe.jpg"],
}),
};
const postResults = await fetch(
"https://app.ayrshare.com/api/post",
optionsPost
).then((res) => res.json());
console.log("Ayrshare says:", JSON.stringify(postResults, null, 2));
};
run();
The response from both ChatGPT and Ayrshare:
ChatGPT says: {
"id": "chatcmpl-6rvrDplRIwdiUURwXzdU3iS0KiYUo",
"object": "chat.completion",
"created": 1678311807,
"model": "gpt-3.5-turbo-0301",
"usage": {
"prompt_tokens": 19,
"completion_tokens": 80,
"total_tokens": 99
},
"choices": [
{
"message": {
"role": "assistant",
"content": "nn"Who needs a cozy bed when you have a shoe! 😺 This little furry feline has found the perfect spot to curl up and take a cat nap. Can you blame them? That shoe looks so snug and comforting. 🥰 What crazy places have you found your cats napping in?" #catsittinginshoes #crazycatnaps #furryfriends"
},
"finish_reason": null,
"index": 0
}
]
}
Ayrshare says: {
"status": "success",
"errors": [],
"postIds": [
{
"status": "success",
"id": "738681876342836_1098688181078083",
"postUrl": "https://www.facebook.com/738681876342836_1098688181078083",
"platform": "facebook"
},
{
"status": "success",
"id": "17987746003883214",
"postUrl": "https://www.instagram.com/p/Cpix-vLtaBq/",
"usedQuota": 1,
"platform": "instagram"
}
],
"id": "KRRormDnpBTgfKYadpmn",
"refId": "d93ca2784c9bf7bf83ce0bf081d16c91598aec28",
"post": "nn"Who needs a cozy bed when you have a shoe! 😺 This little furry feline has found the perfect spot to curl up and take a cat nap. Can you blame them? That shoe looks so snug and comforting. 🥰 What crazy places have you found your cats napping in?" #catsittinginshoes #crazycatnaps #furryfriends"
}
And here are the actual post on Instagram and Facebook:
A New World of Social
We saw how easy it is to generate content and publish to social using a APIs. This is just the beginning of what can’t be done when you combine AI generated content with automated social posting.
If you want to learn more about how ChatGPT works or social media APIs check out these article:
- What Is ChatGPT Doing … and Why Does It Work?
- ChatGPT Explained: A Normie’s Guide To How It Works
- Integrate Social Media APIs Into Your Platform
Generate Social Post and Rewrite Posts
If you’re looking for an endpoint that handles both creating social posts or rewriting post — social networks don’t like duplicate posts — then try out our new ChatGPT powered /generate API endpoint.
The API handles the creation, formatting, adding hashtags & emojis, and even making sure the post is of the requirements length, i.e. Twitter and 280 characters.