Reviews API: Review Management with an API
At most online shopping sites, the power of customer reviews cannot be overstated. They influence buying decisions, shape brand perceptions, and explain customer satisfaction. Recognizing the importance of review management to efficiently handle feedback, Ayrshare presents its Reviews API, designed to streamline the management of reviews across various platforms including Facebook Pages ratings and Google Business Profiles reviews, which are showcased in Google results and Google maps.
Ayrshare’s Social Media API: Simplifying Integration
Ayrshare’s Reviews API is part of its broader social media API, which simplifies the integration of social media management features into your applications. This API is designed to make it easier for developers to incorporate review management functionalities without the hassle of dealing with multiple API integrations. With Ayrshare, you can centrally manage reviews from different platforms, offering a unified approach to review analytics and response strategies.
Features of the Ayrshare Reviews API
The Ayrshare Reviews API stands out for its robust set of features that cater to all aspects of review management:
- Retrieve Reviews: Fetch reviews from multiple platforms, bringing them into a single dashboard for efficient management. The API provides detailed information about each review, including the reviewer’s details, the rating given, and the review content itself.
- Review Analytics: Beyond merely collecting reviews, the API offers analytics capabilities, giving businesses insight into overall customer sentiment with rating averages. This data is useful for understanding customer satisfaction and identifying areas for improvement.
- Reply to Reviews: Engagement is key to maintaining a positive online reputation. The Ayrshare Reviews API allows businesses to directly reply to reviews, fostering a dialogue with customers and demonstrating your commitment to their feedback.
- Delete Replies: In some instances, it may be necessary to delete replies to reviews. The API provides the flexibility to remove responses, ensuring that your review interactions remain appropriate and reflect your brand’s values.
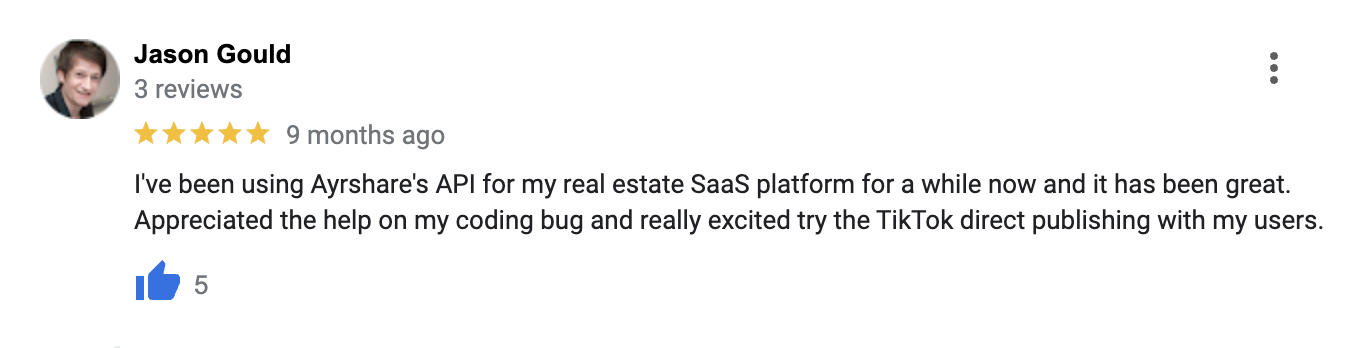
Integrating the Ayrshare Reviews API
Integrating the Ayrshare Reviews API into your application is straightforward. Here’s an example of how you can use the API to fetch reviews using JavaScript. You may get review from either Google Business Profile or a Facebook Page.
const API_KEY = 'API_KEY'; // Replace with your Ayrshare API key
const apiUrl = 'https://app.ayrshare.com/api/reviews?platform=facebook';
const fetchReviews = async () => {
try {
const response = await fetch(apiUrl, {
method: 'GET',
headers: {
'Authorization': `Bearer ${API_KEY}`,
},
});
if (!response.ok) {
throw new Error('Network response was not ok');
}
const data = await response.json();
console.log('Reviews:', data);
} catch (error) {
console.error('Error fetching reviews:', error);
}
};
fetchReviews();
Make sure to replace 'API_KEY'
with your actual Ayrshare API key. Also, note in the URL the query parameter of platform=facebook
to get the Facebook Page rating reviews.
Returned will be the Facebook reviews listing the positive reviews and negative reviews – some will be spammy reviews and others legitimate reviews:
{
"facebook": [
{
"created": "2023-07-11T21:14:41.000Z",
"rating": "positive", // Rating postive, negative
"review": "Makes things easy for connecting social accounts and publishing.",
"reviewer": {
"name": "Sue Smith",
"id": "7283511115003"
}
},
{
"created": "2023-04-19T02:45:05.000Z",
"rating": "positive", // Rating postive, negative
"review": "What an amazing product",
"reviewer": {
"name": "John Smith",
"id": "7501289573254"
}
}
],
"lastUpdated": "2024-02-05T22:47:42.996Z",
"nextUpdate": "2024-02-05T22:58:42.996Z"
}
Replying to a Review
Next, let’s look at how your business can actively manage your customer interactions with examples of replying to a review and deleting a review reply. These endpoint operations are crucial for maintaining an engaging and responsive online presence.
Replying to a review using the Review API requires the reviewId
(from the get reviews call above) and the reply message. Here’s a JavaScript example demonstrating how to post a reply to a Google Business Profile review:
const API_KEY = 'API_KEY'; // Replace with your Ayrshare API key
const reviewId = 'Review Id'; // Replace with the ID of the review you want to reply
const apiUrl = `https://app.ayrshare.com/api/reviews/reply`;
const replyToReview = async () => {
try {
const response = await fetch(apiUrl, {
method: 'POST',
headers: {
'Authorization': `Bearer ${API_KEY}`,
'Content-Type': 'application/json',
},
body: JSON.stringify({
platform: "gmb", // Google Business Profile
reviewId: reviewId,
reply: "Thank you for your feedback! We're glad you enjoyed our service.",
}),
});
const data = await response.json();
console.log('Reply success:', data);
} catch (error) {
console.error('Error replying to review:', error);
}
};
replyToReview();
In this Google reviews example, you’ll need to replace 'API_KEY'
with your actual Ayrshare API key and 'Review Id'
with the ID of the review you’re replying. This function sends a POST request to the Ayrshare API, adding your reply to the specified review.
Deleting a Review Reply
There might be instances where you need to delete a reply to a review. Whether it’s due to a typo or a change in response strategy, the Ayrshare Reviews API also supports deleting replies. Here’s how you can do it for Google reviews:
const API_KEY = 'API_KEY'; // Replace with your Ayrshare API key
const reviewId = 'Review Id'; // Replace with the ID of the review which contains the reply
const apiUrl = `https://app.ayrshare.com/api/reviews`;
const deleteReviewReply = async () => {
try {
const response = await fetch(apiUrl, {
method: 'DELETE',
headers: {
'Authorization': `Bearer ${API_KEY}`,
'Content-Type': 'application/json',
},
body: JSON.stringify({ platform: "gmb", reviewId }),
});
const data = await response.json();
console.log('Delete reply success:', data);
} catch (error) {
console.error('Error deleting review reply:', error);
}
};
deleteReviewReply();
For this operation, you’ll need to replace 'API_KEY'
with your Ayrshare API key and 'Review Id'
with the ID of the reply you intend to delete. This script sends a DELETE request to the Ayrshare API to remove the selected reply.
Unfortunately no social networks allow you to leave reviews or remove reviews, only reply to reviews that someone else has left.
These examples illustrate the flexibility and control Ayrshare’s Reviews API offers businesses in managing their online reviews. By integrating these capabilities, companies can ensure their review management process is as dynamic and responsive as their customer service, fostering positive relationships and a strong online reputation.